This lesson is about the numeric functions in VB6. Before proceeding with this lesson, you may want to read VB6 Tutorial 34: Handy Numeric functions.
As mentioned in the previous lesson 34, VB6 contains many useful operators and functions to efficiently work with numbers. In this post, I’ll go over a few more of them.
Abs
It returns the absolute value of a number.
Example
Private Sub Form_Load() Form1.Show Print abs(-10) End Sub
Output
10
Sin, Cos and Tan
These functions return the sine, cosine and tangent of an angel. These functions receive arguments in radian. Convert radian to degree multiplying Pi/180. You may declare a Pi constant at the beginning.
Example
Private Sub Form_Load() Form1.Show Print Sin(90) Print Cos(0) Print Tan(45) End Sub
Output
0.893996663600558
1
1.61977519054386
Exp
The Exp function returns e (the base of natural logarithms) raised to a power.
Example
Private Sub Form_Load() Form1.Show Print Exp(5) 'equivalent to e^5 End Sub
Output
148.413159102677
Log
The Log function returns the natural logarithm of a number.
Example
Private Sub cmdPrint_Click() Print Log(10) End Sub
Output
2.30258509299405
Sgn
This function indicates the sign of a number. It returns -1, 0 or 1 if the number is negative, zero or positive respectively.
Example
Private Sub cmdShowSign_Click() Print Sgn(-10) End Sub
Output
-1
The Fix function
Fix function truncates the decimal part of a number. In Lesson 34, you have seen that the Int function also truncates the decimal part of a number. But this function is different from the Fix function. You see the difference when the number is negative in case of the Int function. So the function that actually truncates the decimal part of a number is Fix.
Example
Private Sub cmdTruncate_Click() Print Fix(4.5) Print Fix(-4.5) End Sub
Output
4
-4
The Rnd function
Sometimes, we need to generate some random values. The Rnd function returns a random value between 0 and 1.
QuickHint:
Rnd is not a variable, it is a function.
Example
Each time the CommandButton is clicked , it gives a different value between 0 and 1.
Private Sub cmdGenerateRandomNumbers_Click() Randomize() 'Randomizes the number lblResult.Caption = Rnd End Sub
Output
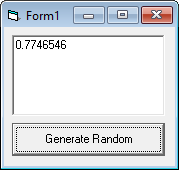
The attached example project demonstrates how to generate values between the numbers you specify.
The Hex and Oct functions
The Hex function returns the string representation of its hexadecimal equivalent and the Oct function converts the number to Octal.
Example
Private Sub cmdConvert_Click() Print Hex(10), Oct(50) End Sub
Output
A 62
To convert a hexadecimal or octal number into decimal value
Example
Private Sub cmdConvert_Click() Print Val("&H" & "A") 'A is the Hexadecimal number Print Val("&O" & "62") '62 is an octal number End Sub
Output
10
50